Because of two current events this is my contribution to the Coronavirus COVID-19 and the #MonthOfMaking from the Raspberry Pi Foundation. If you can kill two birds with one stone, then you should do it, right? Since we are all doomed anyway, it’s better to publish the article as soon as possible. Soon nobody will be able to read it anymore. Friday is also the 13th! We are really f*cked this time …
My friend from Australia told me about hamster or panic buying in Australia. A woman bought 196 rolls of toilet paper. In some supermarkets there are now supervisors in charge of the toilet paper so that everybody can only buy a certain amount. 196 rolls — so even if you shit to use one roll a day … the panic is worse than the virus, it seems to me.
Where do I get the data from?
I get the updates for the data from https://covid19.mathdro.id/api, respectively I want to have the data for the UK and therefore I use the URL https://covid19.mathdro.id/api/confirmed. The result will look like this:
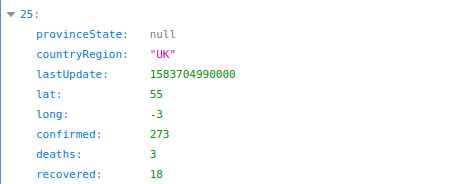
To query the URL, I use cfscraper. I don’t know if it is necessary in this case, but with my Bitcoin tracker I need it, otherwise Cloudflare will interfere. You can install it via PIP. If it is not available, install it:
sudo apt install python3-pip
After that use that command:
pip3 install cfscrape
You can also check if the package for the Sense HAT ist already installed:
Now I have all the components to get the data. My program looks like this — I only query the data from the UK and display it on my Sense HAT.
from sense_hat import SenseHat
import json
import cfscrape
sense = SenseHat()
scraper = cfscrape.create_scraper()
blue = (0, 0, 255)
yellow = (255, 255, 0)
red = (255, 0, 0)
green = (0, 255, 0)
black = (0, 0, 0)
url = 'https://covid19.mathdro.id/api/confirmed'
cfurl = scraper.get(url).content
data = json.loads(cfurl)
infectedUK = data[25]['confirmed']
deathsUK = data[25]['deaths']
recoveredUK = data[25]['recovered']
sense.set_rotation(90)
sense.show_message(str(infectedUK), text_colour=yellow, scroll_speed=0.1)
sense.show_message(str(deathsUK, text_colour=red, scroll_speed=0.1)
sense.show_message(str(recoveredUK), text_colour=green, scroll_speed=0.1)
sense.clear()
Confirmed infections are shown in yellow. There are currently 3 confirmed deaths in the UK and therefore the Sense HAT is displayed as a red 3. Recovered people are displayed in green.
You can customize the script as you like. Maybe you want to have another country, or several countries, or even the worldwide data.
Update: Data changes
In case the countries change number you could actually query for UK. The script would change like that:
from sense_hat import SenseHat
import json
import cfscrape
sense = SenseHat()
scraper = cfscrape.create_scraper()
blue = (0, 0, 255)
yellow = (255, 255, 0)
red = (255, 0, 0)
green = (0, 255, 0)
black = (0, 0, 0)
url = 'https://covid19.mathdro.id/api/confirmed'
cfurl = scraper.get(url).content
data = json.loads(cfurl)
for val in data:
if val['countryRegion'] == 'UK':
infectedUK = val['confirmed']
deathsUK = val['deaths']
recoveredUK = val['recovered']
sense.set_rotation(90)
sense.show_message(str(infectedUK), text_colour=yellow, scroll_speed=0.1)
sense.show_message(str(deathsUK), text_colour=red, scroll_speed=0.1)
sense.show_message(str(recoveredUK), text_colour=green, scroll_speed=0.1)
sense.clear()
Now you would get the data for UK regardless where it is.