I love my Sense HAT. You can do so much with it — even build your own weather station. Now I thought about that you can also create a Bitcoin price tracker with it. Of course, it can also be used for other crypto currencies. It worked, but there were a few pitfalls. I would like to describe here the way to my solution: how to get the Bitcoin price with the Raspberry Pi and Python 3 and show it on the Sense HAT.
Probably programming gurus are packing my project into a one-liner. But I do not program that much and my solution works. I am satisfied with it. I go through what I have done step by step. You can find the complete script in the middle of the article.
Get Bitcoin price with Python and Raspberry Pi
There are now several websites that can be used. I chose cryptonator.com because it delivers the needed values in JSON format. Furthermore, the provider supports many currencies and crypto currencies and you can combine as you like. Even a Euro-Dollar pair is possible. Moreover, you get values from several exchanges and if you want, you can display the value from a specific exchange.
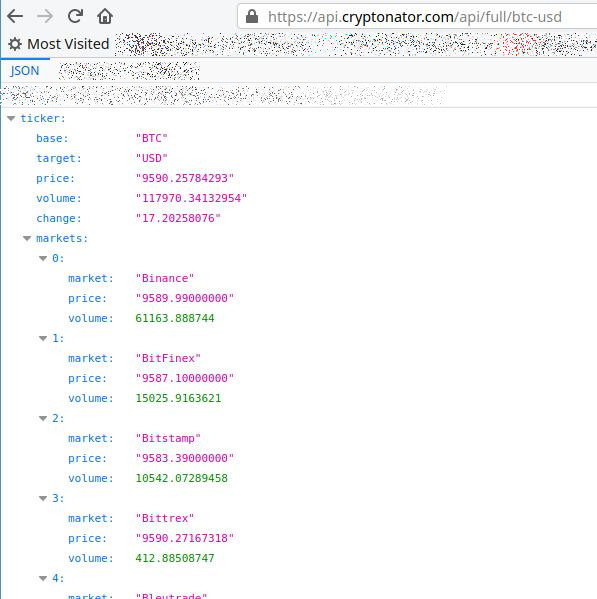
For me the ticker is enough therefore I will use the following URL (the difference is that in the URL full is exchanged for ticker):
https://api.cryptonator.com/api/ticker/btc-usd
So I get the same output as in the screenshot, but without the markets. If I get the URL via Python’s requests, I bump into the first problem.
Cloudflare doesn’t like this
I wanted to get the JSON data and got an error message.
json.decoder.JSONecodeError: Expecting value: line 1 column 1 (char 0)
Now I had a look at what I was actually getting from the website and this is what came out:
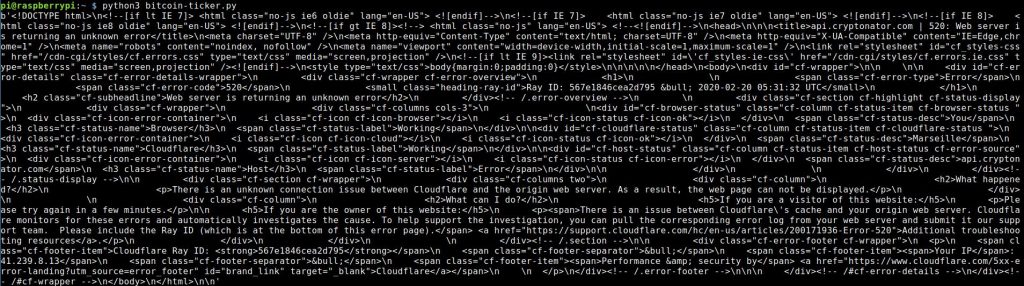
In other words, Cloudflare does a bot check and therefore I don’t get the data I want. But a request with curl worked. Now there are two options.
- Fetch the data with curl and process it further
- I use cfscrape — I found this after some research
The latter is a simple Python module that bypasses the anti-bot check of Cloudflare. It seems more elegant at this point and that’s why I decided to use it. You install the module on the Raspberry Pi via pip. You may have to install the program first:
sudo apt install python3-pip
After that
pip3 install cfscrape
Now I get the data, can process the JSON format and check the price. Since I do not need the price after the dot, I only take the integer:
import json
import cfscrape
scraper = cfscrape.create_scraper()
url = 'https://api.cryptonator.com/api/ticker/btc-usd'
cfurl = scraper.get(url).content
data = json.loads(cfurl)
price = data['ticker']['price']
rounded = price.partition('.') # The dot splits it
print(rounded[0]) # Price as integer
That’s it — all the magic done. As described here, you can query the Bitcoin price with Raspbian on the Raspberry Pi or any system with Python. Now that I have the value, I can display it on the Sense Hat or process it on the Raspberry Pi as I like.
Showing the Bitcoin price on the Sense HAT
From here on, the sky’s the limit. I have decided, in line with the current situation, to display the value in red when Bitcoin is below $10,000 and green when it breaks the psychologically important mark again.
Therefore, I also define the colors for the Sense HAT and import the library, of course. I need the line with the rotation because my Sense HAT hangs on the wall. The whole script looks like this:
from sense_hat import SenseHat
import json
import cfscrape
scraper = cfscrape.create_scraper()
sense = SenseHat()
blue = (0, 0, 255)
yellow = (255, 255, 0)
red = (255, 0, 0)
green = (0, 255, 0)
url = 'https://api.cryptonator.com/api/ticker/btc-usd'
cfurl = scraper.get(url).content
data = json.loads(cfurl)
price = data['ticker']['price']
rounded = price.partition('.')
sense.set_rotation(90) #optional my Sense HAT hangs that way on the wall
if int(rounded[0]) >= 10000:
sense.show_message(rounded[0], text_colour=green, scroll_speed=0.1)
else:
sense.show_message(rounded[0], text_colour=red, scroll_speed=0.1)
sense.clear() # if you want to delete the display of the Sense HAT
Possible variations
As an idea, you could use red and green like the stock markets, indicating whether the value has changed downwards or upwards. You get the value from change:
data['ticker']['change']
If it is negative, it went down (red). If it’s positive, we show it green. The end of the script could look like this:
if float(data['ticker']['change']) >= 0:
sense.show_message(rounded[0], text_colour=green, scroll_speed=0.1)
else:
sense.show_message(rounded[0], text_colour=red, scroll_speed=0.1)
sense.clear()
Or you can show the value, but change the background depending on the change. You can change the background color of the Sense HAT with back_colour — I defined the color black for that — black = (0, 0, 0):
if float(data['ticker']['change']) >= 0:
sense.show_message(rounded[0], text_colour=black, back_colour=green, scroll_speed=0.1)
else:
sense.show_message(rounded[0], text_colour=yellow, back_colour=red, scroll_speed=0.1)
sense.clear() # If you don't use it the display will stay lit
I prefer not to show the value in binary form on the display of the Sense HAT. Sooner or later there won’t be enough space left. 😉
What are your ideas? Let me know!
Display Bitcoin price every 5 minutes on the Sense HAT
For example, if you want to have the Bitcoin price flicker above your Sense HAT every 5 minutes — easy. I assume you have saved the code in a file called btc-ticker.py and it is located in the directory /home/pi. Now create a cronjob on your Raspberry Pi. First open the crontab with the following command:
crontab -e
Then you add a line like this:
*/5 * * * * /usr/bin/python3 /home/pi/btc-ticker.py >> /dev/null 2>&1
The command is executed every 5 minutes. For example, 2 pm, 2:05 pm, 2:10 pm so on.